참고
vuex import 스타일 - https://kdydesign.github.io/2019/05/09/vuex-tutorial/
vuex 개념 - https://joshua1988.github.io/web-development/vuejs/vuex-actions-modules/
할일- 스토어 폴더 파일 구조 변경팁
1. main.js에 store 등록했는지 확인
2. 여러개 store 사용할때 모두 module화 안하면 namespaced 인식 못함
3. createNamespacedHelpers 사용할거면 모듈에서 namespaced : true 하기
Vuex store 여러개 사용하기
1. store 폴더구조 정하기
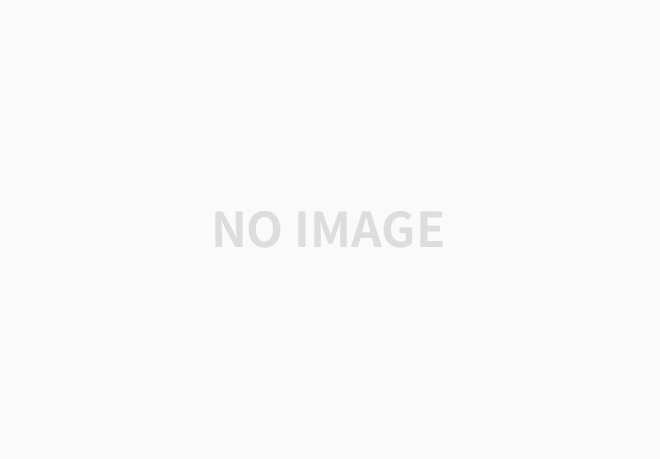
2. 각 기능마다 state, mutations, getters, actions 모듈화해서 export 하기
// weatherData.js(날씨 데이터 모듈)
var axios = require('axios')
var cheerio = require('cheerio')
const state = {
nowTemperature: '12', // 현재 온도
nowWeatherCheck: '흐림', // 맑음, 흐림 체크
todayMin: '1', // 최저기온
todayMax: '15', // 최고기온
todayDust: '2', // 미세먼지
todayDDust: '2', // 초미세먼지
// test: 'testsadasdwe',
}
// vue의 computed와 비슷
const getters = {
}
// state를 수정할 때 사용
const mutations = {
SET_TEMPERATURE(state, data) {
state.nowTemperature = data
},
SET_WEATHERCHECK(state, data) {
state.nowWeatherCheck = data
},
SET_TODAYMIN(state, data) {
state.todayMin = data
},
SET_TODAYMAX(state, data) {
state.todayMax = data
},
SET_TODAYDUST(state, data) {
state.todayDust = data
},
SET_TODAYDDUST(state, data) {
state.todayDDust = data
},
}
// 비동기를 사용할 때, 또는 여러 mutations를 연달아 송출할 때
const actions = {
getData({state, commit}, data) {
axios.get('https://search.naver.com/search.naver?sm=top_hty&fbm=1&ie=utf8&query=%EB%82%A0%EC%94%A8')
.then(res => {
var $ = cheerio.load(res.data)
commit('SET_TEMPERATURE', $('#main_pack > div.sc.cs_weather._weather > div:nth-child(2) > div.weather_box > div.weather_area._mainArea > div.today_area._mainTabContent > div.main_info > div > p > span.todaytemp').text())
commit('SET_WEATHERCHECK', $('#main_pack > div.sc.cs_weather._weather > div:nth-child(2) > div.weather_box > div.weather_area._mainArea > div.today_area._mainTabContent > div.main_info > div > ul > li:nth-child(1) > p').text())
commit('SET_TODAYMIN', $('#main_pack > div.sc.cs_weather._weather > div:nth-child(2) > div.weather_box > div.weather_area._mainArea > div.today_area._mainTabContent > div.main_info > div > ul > li:nth-child(2) > span.merge > span.min').text())
commit('SET_TODAYMAX', $('#main_pack > div.sc.cs_weather._weather > div:nth-child(2) > div.weather_box > div.weather_area._mainArea > div.today_area._mainTabContent > div.main_info > div > ul > li:nth-child(2) > span.merge > span.max').text())
commit('SET_TODAYDUST', $('#main_pack > div.sc.cs_weather._weather > div:nth-child(2) > div.weather_box > div.weather_area._mainArea > div.today_area._mainTabContent > div.sub_info > div > dl > dd:nth-child(2)').text())
commit('SET_TODAYDDUST', $('#main_pack > div.sc.cs_weather._weather > div:nth-child(2) > div.weather_box > div.weather_area._mainArea > div.today_area._mainTabContent > div.sub_info > div > dl > dd:nth-child(4)').text())
})
},
}
export default {
namespaced:true,
state,
mutations,
actions,
getters,
};
// spanishWord.js(스페인어 데이터 모듈)
//vue의 data와 비슷
const state = {
getWord: 'hellofe3ssss4324sㄴㄴㄴㄴ'
}
// vue의 computed와 비슷
const getters = {
}
// state를 수정할 때 사용
const mutations = {
// [GET_WORD](state, data) {
// state.getWord.push(data)
// }
}
// 비동기를 사용할 때, 또는 여러 mutations를 연달아 송출할 때
const actions = {
}
export default {
namespaced:true,
state,
mutations,
actions,
getters,
};
3. index파일(중앙 저장소)에 모듈화 한 파일들 import하기
// index.js(중앙 저장소)
import Vue from 'vue'
import Vuex from 'vuex'
import wordStore from './wordStore/spanishWord'
import weatherStore from './weatherStore/weatherData'
Vue.use(Vuex)
export default new Vuex.Store({
modules: {
spanish: wordStore,
weather: weatherStore,
}
})
4. 해당 컴포넌트에서 import후 화면에 뿌려주기
기본 vuex 사용 할 때
// main.vue
import store from './store'
export default {
name: 'main',
store,
components:{
'mainTitle': mainTitle,
'nowWeather': nowWeather,
},
computed:{
nowTemperature() {
return this.$store.state.nowTemperature
},
nowWeatherCheck() {
return this.$store.state.nowWeatherCheck
},
todayMin() {
return this.$store.state.todayMin
},
todayMax() {
return this.$store.state.todayMax
},
todayDust() {
return this.$store.state.todayDust
},
todayDDust() {
return this.$store.state.todayDDust
},
// test() {
// return this.$store.state.test
// }
},
data() {
return {
}
},
methods: {
}, // methods 끝
}
</script>
mapState, mapActions 사용 할 때
// main.vue
<script>
import mainTitle from './main/mainTitle'
import {createNamespacedHelpers} from 'vuex'
const nowWeather = createNamespacedHelpers('weather')
export default {
name: 'main',
components:{
mainTitle
},
computed:{
...nowWeather.mapState([
'nowTemperature',
'nowWeatherCheck',
'todayMin',
'todayMax',
'todayDust',
'todayDDust',
])
},
data() {
return {
}
},
methods: {
...nowWeather.mapActions([
'getData'
])
}, // methods 끝
mounted() {
this.getData()
}
}
</script>
'Vue-프로젝트' 카테고리의 다른 글
vue-프로젝트 단어장(17일차) (0) | 2020.04.28 |
---|---|
vue-프로젝트 단어장(16일차) (0) | 2020.04.27 |
vue-프로젝트 vuex(14일차) (0) | 2020.04.23 |
vue-프로젝트 (13일차) (0) | 2020.04.22 |
vue-프로젝트 코인 차트 만들기(12일차) (0) | 2020.04.21 |